What is a delegate?
- The delegate is a type that holds a reference to a method similar to function pointers.
- The delegates allow other methods to be passed as parameters.
- The method return type can be different from the delegate type.
- It is mainly used for implementing events and call-back methods.
- The delegate is derived from System. Delegate class.
What is an event?
- An event is an occurrence of actions such as button clicks, mouse movements, etc.
- Events are declared, raised, and associated with delegates or events handlers in the same or other classes.
- The class object that invokes an event and notifies other objects is known as the publisher.
- The class which receives the notification or object which accepts the event and includes an event handler known as a subscriber.
For more such programming videos check the link
Need For delegates and events in c#
Any static or instance method that matches delegate type can be assigned to the delegate. This provides a convenient way to change the method call or add new code programmatically into the existing classes.
The delegates reduces coupling between the publisher and subscriber since the other methods and properties of the object implementing method is not accessible to the caller.
Declaration of delegates and events in c#
Declaring a delegate
Syntax:
delegate return-type delegate-name(parameters);
Delegate instantiation:
- After declaring the delegates it is necessary to create a delegate object and associate it with a method.
- The delegate object is created using the new keyword.
- Similar to function call other methods can be passed as an argument while creating instances.
public delegate void display(string s)
public void sub(string){…}
display d2=new display(sub);
Declaring an event:
Declare delegate type for the event:
public delegatedata-type delegate-name(parameters);
Declare event using event keyword for delegate variable:
event delegate-name event-name;
Example for Delegates and Events in c#
To understand the events and delegates better, let us consider an example
A youtube channel and a subscriber, whenever a new video is uploaded in the channel, the subscriber gets notification about the new video uploaded in the channel.
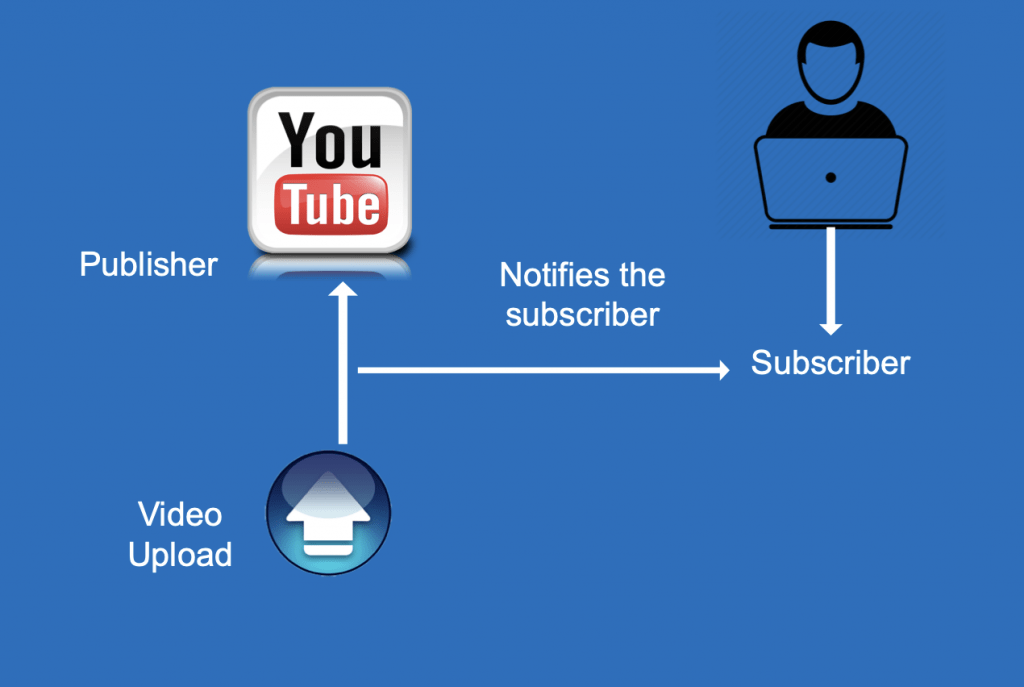
Here the youtube channel is the publisher and the video upload is the event and the event handler method will be the GetNotified method in the subscriber class.
Now let us create a simple console application in the visual studio
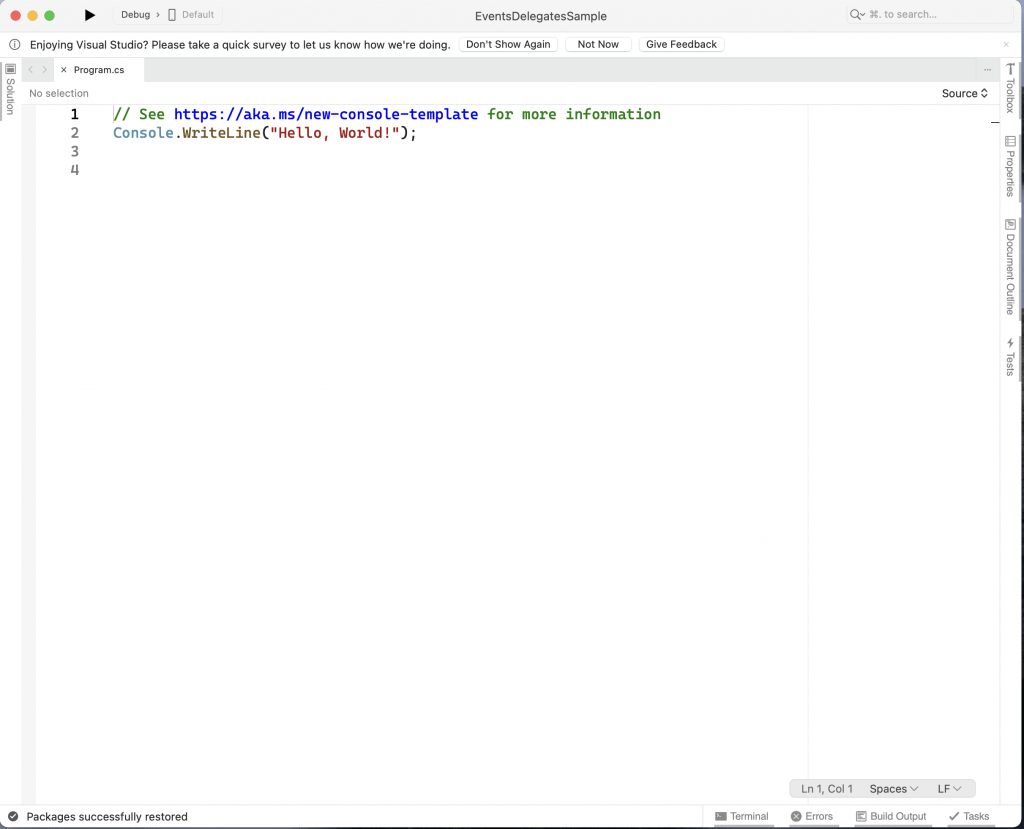
For .net framework versions over 6.0, the Program.cs file looks like this, a new program style. The compiler handles all the creation such as Program class and Main methods, we just need to include only necessary top level statements.
If you are not comfortable with new program style, you can create old program style Program.cs file by selecting the check box as shown below, while creating the application.
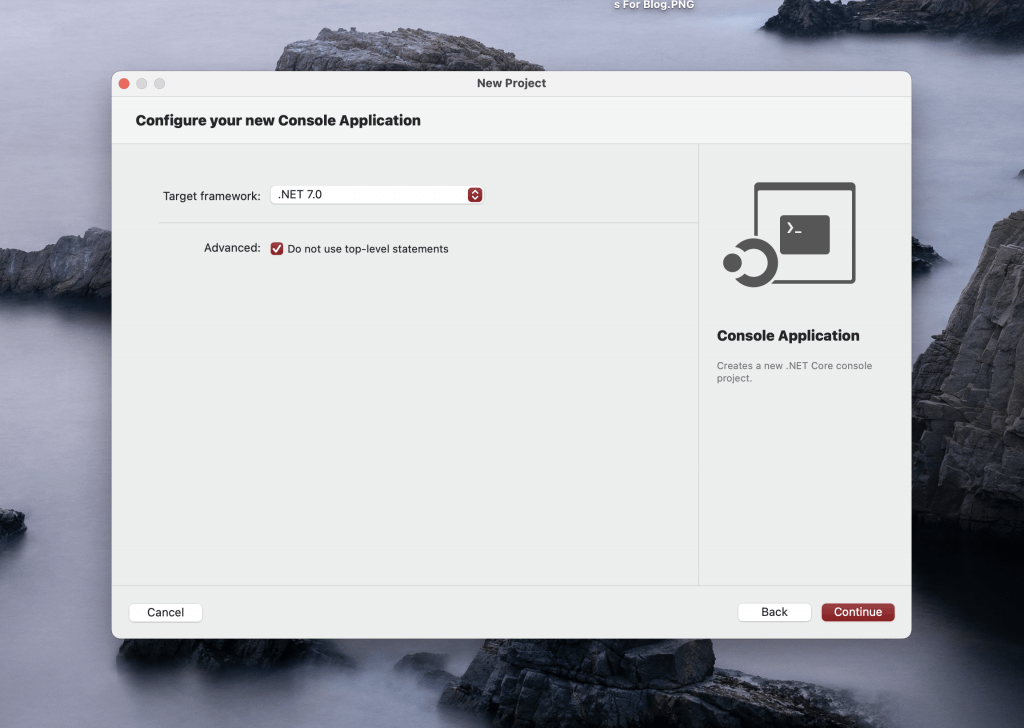
Now if you create the project, you will get the older program style Program.cs file as shown below:
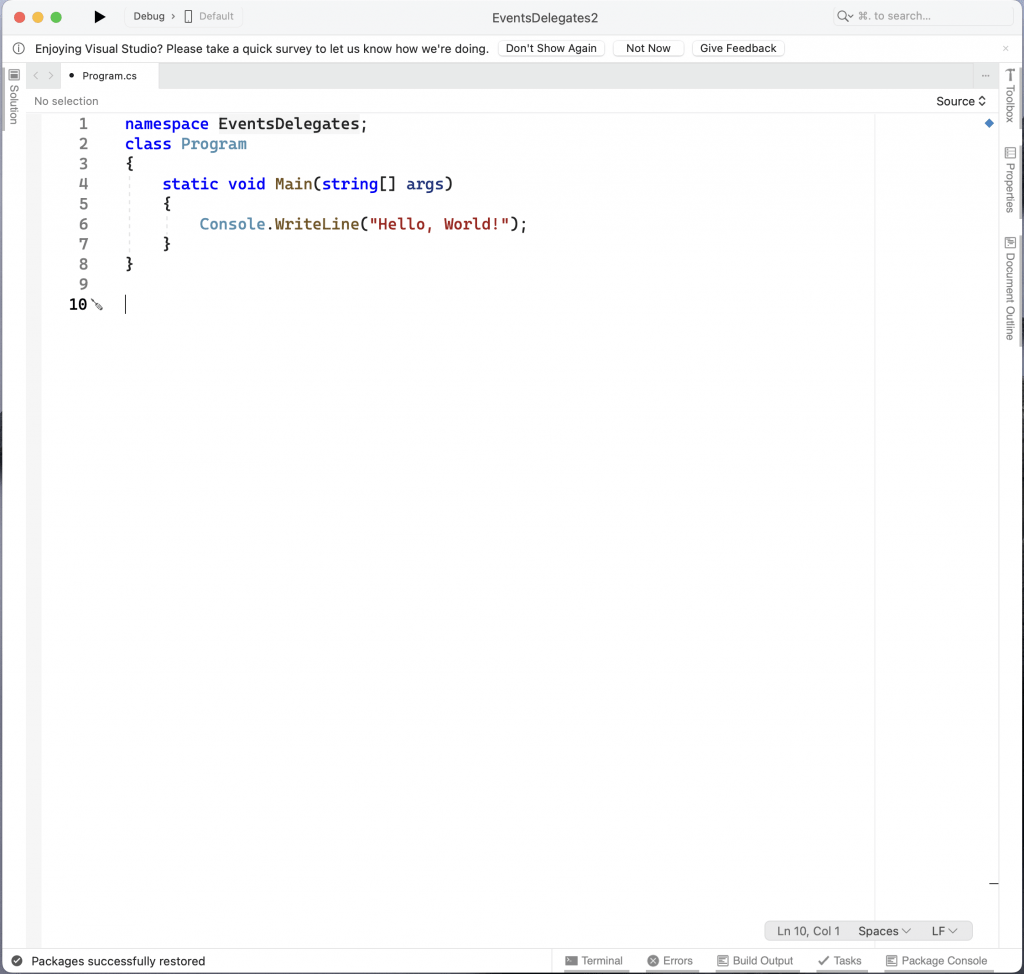
Now we need two classes, a publisher class to implement or raise the event and a subscriber class to receive the event.
Publisher Class
In Publisher class, we have to implement the event, to do that we have to include 3 steps
- Declare a delegate
- Declare an event
- Raise an event.
In the above code, we have SubscriptionHandler which is the EventHandler that accepts string parameters. We create an event(Subscribed) for the SubscritptionHandler.
It includes a video upload method that just displays a VideoUploaded message and calls the publisher method (OnSubscribed) that invokes the event handler method. Since it is suggested to name the method that raises the event to prefix the method with On.
Subscriber Class
In the Subscriber class, we must include the GetNotified method to receive an event.
The GetNotified method in the subscriber class must be compatible with the signature of the delegate. It receives the title of the video and displays the title along with the string uploaded. Now we have created both the Publisher class and Subscriber class, now in the Program.cs file we have to register the handler for the event.
We create instances for both the classes and register handler for event using the convention +=, and even though GetNotified is a method, no parenthesis is used. It acts like a reference to a method or function pointer. Then the video upload method is called which produces the following result.
EventHandlers in C#
In .net we have delegate-type EventHandlers in two forms
- To create a custom event data class, derive EventArgs base class.
- Built-in delegate types such as EventHandler are used for events that do not include event data
- EventHandler<TEventArgs> which includes data.
VideoDetailEventArgs Class
To pass video details as parameters to the SubscriptionHandler we can create a custom class VideDetailEventArgs that inherits EventArgs class as shown below
In the delegate declaration, we include two parameters, sender information, and detail about the event args. Since the method in the subscriber class must be compatible with the type of parameters passed in the delegate, we pass the sender(this-present class) and videodetails as arguments.
The GetNotified method in the subscriber must receive the two parameters passed.
Built-in Event Handlers:
Instead of declaring an event with our own delegate, we can include a built-in Event handler with a parameter of type VideoDetailEventArgs as given below
public event EventHandler(VideoDetailEventArgs) Subscribed;
If no parameter needs to be passed, we can simply use EventHandler
public event EventHandler Subscribed;
Multicast Delegates
- Only delegates of the same type can be composed.
- The ‘+’ operator is used to compose a delegate object.
- The ‘-’ operator is used to remove a delegate from a composed delegate.
- The composed delegate calls two individual component delegates.
- During delegate invocation, multiple methods can be called known as multicasting.
To know much more about events and delegates check the Microsoft official documentation link