In addition to programming skills, all developers must be aware of two important topics Git and GitHub which help very much, especially in the present scenario where many of us are working remotely.
In such a situation many developers will be working on different portions of a project such as a Login page, payment page, settings page, etc. Any modifications or changes made by one developer must be available for others, to complete the project without any errors, to handle such a situation, we use Git.
Git and GitHub are the terms many think as the same but actually, they are two different technologies we will learn more about this in this article.
What is Git?
Git is an open-source distributed Version Control System created by Linus Torvalds in 2005 to develop the Linux kernel.
The VCS helps us to track changes made in the file, programs, or other collection of information. It retains and reverts back to the previous state of the file, makes a copy, new code changes, and allows us to merge the copy with the original file in the future.
The VCS maintains a snapshot of every change made to the project and saves a copy. Some of the VCS tools other than git are Subversion, Concurrent Versioning System(CVS), and Mercurial.
Git Installation:
To use Git, it must be installed on your computer, to do this you can download the latest version of Git from the official website. If you have git already installed, check the version on your PC using git –version.
Features of Git:
Distributed:
- Allows multiple developers to work remotely on the same project without interfering with other developer’s code.
- The developer can have the local copy of the development history and changes copied from one repository to another.
Compatible:
- It is compatible with all the existing operating systems.
- It can also access other version control tools such as SVN(), and SVK, so they can easily migrate to git without copying files from other VCS.
- CVS server emulation feature allows existing CVS clients and IDE plugins to access git repositories.
Non-Linear Development:
- Git stores the current state of the project by creating a tree structure that is a non-linear data structure.
- It supports seamless branching and merging which helps in navigating and visualizing a non-linear development.
Branching:
- Git provides a feature called branching that helps us to make changes in the file without affecting the original project.
- Multiple local branches can be created and merged later.
- The master branch contains the production quality code.
Light Weight:
- The data that is stored from the central to the local repository can be huge, git handles this situation using a compression technique.
- The compression technique compresses the data and stores it in the local repository occupying less space.
Speed:
- Fetching data from the local repository is very much faster than the remote repository.
- Git is very much faster than other VCS tools since it is written in C language which is close to machine languages.
Open Source:
- Git is created as an open source so that the users can create and modify the source for free.
- This enables users with important features such as repository space, code privacy, speed, and accuracy which are given as paid features in other VCS tools.
Secure:
- It is highly secure since it uses a (SHA1)secure hash function to identify objects in the repository.
- All files are checked and checksums are retrieved at the time of checkout.
- SHA-1 is cryptographic and uses message direct algorithm md4 and md5 that converts commit object into 40-digit hexadecimal code.
Repository:
- A directory or storage space where all the project files are stored is known as a repository.
- It can be a local file on a computer or a storage space on GitHub or any other host where all codes and images can be stored.
- The two types of repositories are
- Central repository and
- Local repository.
Central Repository
- It is located on a remote server.
- It includes “.git” repository folder.
- The team can share and exchange files.
Local Repository
- It is located on a local machine.
- It resides as a “.git” file inside the root.
- Only admin can work.
Git operations & Commands
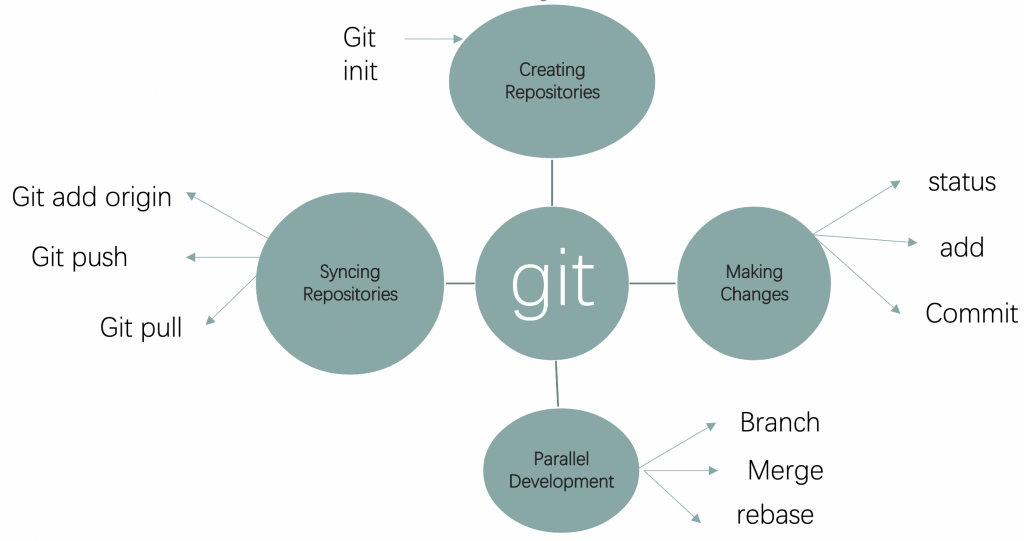
Git Commands:
To start with git commands, the foremost thing we need is a local repository. As we already know the local repository is the local copy of the repository where all files are present.
The files can be accessed by only the admin and can do all changes such as adding a file, removing a file, modifying a file, committing changes, etc.
Initialize Git:
This command is used to change a directory into an empty repository. First, open your terminal and create a directory using
mkdir folder-name
move to the created folder using the following command
cd folder-name
now use the following git command to initialize an empty git repository
git init
Git Command to Add Files
To add files to the staging area, the git add command is used.
All the files that are added and modified are not committed, we must add files to the staging area or git index, then we can make a commit.
First, we create an empty file using the following command,
touch filename
and we edit the file and include some code to the empty file using the command,
gedit filename
save changes and type the following command to add the file to the staging area.
git add file-name
To move multiple files from the local repository to the staging area the following command is used.
git add .
Git Command to Commit Files:
Committing the files in the staging area records all the changes made to the files in the local repository. Each commit includes a unique ID and it is always a good practice to add a message to each commit for future reference.
git commit -m "commit message"
Git Command to get state:
To get the current state of the working directory the following command is used.
git status
It helps to find the untracked, stages, or unstaged files in the directory. If you add a new file and make changes but if not added, you will get untracked files.
Checking status after adding gives the message, that “no changes added to the commit”.
After committing when you check the status, it shows “nothing to commit”.
Configuring fields using Git command:
The various fields in git such as username, emailid, aliasname, text editor and visualization tools can be set using the following configuration command.
git config
The configfuration can be done for different levels of git such as local, global and system level configuration.
We can view the values of the local git repository using the command
git config --list --local
and global settings using the following command
git config --list --global
System level settings using
git config --list --system
we can change the username and password for all directories of a user using
git config --global user.name "user-name"
git config --global user.email "emailid"
We can set the text editor for entering data using the command
git config --global core.editor "editor"
Using the following command shortens lengthy commands with alias name
git config --local alias.co checkout
git config --local alias.c commit
To compare files that raise conflicts while merging we can use
git config --global merge.tool <merge-tool-name>
Git command for branching:
It is one of the important feature available in modern version control systems. To add a new feature, fix a problem or make some changes without affecting the original code, we can create a new branch. It acts as a pointer that tracks all changes made and maintains and cleans up unwanted changes before merging to the master branch.
In this we will learn about creating a branch, merging, and switching branches.
We create a new branch and switch to the branch using a single command
git checkout -b branch-name
or create branch using
git branch branch-name
To switch to the new branch following command can be used
git checkout branch-name
Now you can make necessary changes using
gedit file-name
Add files to the staging area
git add .
Commit the files in the staging area using the following command
git commit -m "message"
After committing files in the staged area, switch to the master branch
git checkout master
and merge the branch using
git merge branch-name
Once merged, the branch can be deleted using
git branch -d branch-name
git branch -D branch-name
The first command is preferred over the second since -D will delete even without notifying any errors if not merged.
Working with the remote repository:
To connect with a remote repository use the following command. Create your new repository in GitHub and select the clone or download button, you will get an ssh key copy and include it in the command.
git remote add origin sshlink
Now to create a local copy of the files in the remote repository, we need to clone.
To do that create a directory and move to the created folder refer to commands in initialize git and then clone using the command
git clone sshlink
To check files in the folder use the following command
ls
Suppose if you make some changes in the files of the remote repository, we must use the pull command to pull the committed changes in the remote repository.
git pull origin master
If we create a new file or make some changes in the local repository, we need to use the push command to push committed changes to the remote repository.
git push origin master
Advanced Git Commands:
- When some changes are made to files in the local repository, but not committed since we have some other important problem to handle, we can use the following command.
git stash -u
The above command helps to store those files and provide clean working directory.
To list the modifications stored by stash command we can use the following command.
git stash list
To check the files in the list we use,
git stash show
Again when we are ready to commit changes we can use the command
git stash apply
2. If you want to see the commit history for a repository such as author, date and commit values, we use log command
git log
git log --author=author-name #filter commits of particular author
git log --since=data #filter commits of specific duration
git log --reverse # show commit history in reverse order
git log --stat commitvalue #view summary of file
git log --oneline #To see list of commits
3. Once you have made some changes and committed a file, if you want to revert back the commit, you can use
git revert commitvalue #commit value can be found using git log --oneline
git revert HEAD #Reverts back the last commit changes to original
4. To integrate changes from diffrent branches and makes linear sequence of commits so that commit history and directory looks clean.
git rebase branch-name
git rebase --skip
git rebase --continue
git rebase --abort
In this article, we learnt all the important git commands, to know more about commands with parameters, check the official documentation of git at Git – Reference (git-scm.com)
Top ,.. top top … post! Keep the good work on !
Sure, Thank you