Need For MVVM
In Multiplatform application development, the UI is mainly created with XAML, and the code behind C# code handles the behaviors of the UI. This tight coupling between the design and business logic creates complex maintenance issues such as increased UI modifications cost and difficulty in unit testing.
The Model View ViewModel(MVVM) maintains a clear separation between the UI and business logic using the view and ViewModel structure. This helps with easier testing, modification, maintenance, and code reusability. It also provides a way for designers and developers to work on their parts efficiently and collaboratively.
MVVM pattern
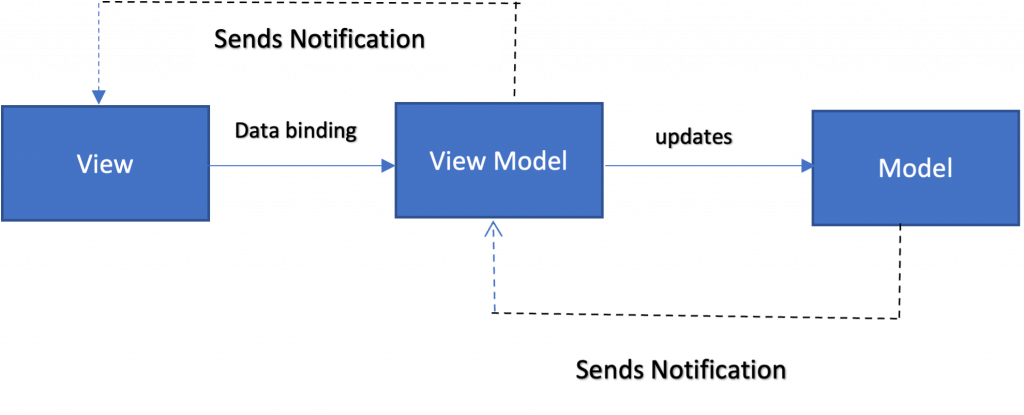
It is necessary to understand the responsibilities of each part – Model, View, and ViewModel of the MVVM pattern.
View
The view defines the structure, layout, and appearance of the screen which is visible to the user. It is usually defined in XAML but in some complex cases, the code behind handles the UI design.
It just includes only UI design and necessary initializations and does not include anything about business logic. Instead of code behind ViewModel handles enabling and disabling UI elements and handling events such as button click and item selection.
A view derives from either ContentPage or ContentView and is represented by using data templates. The data template binds to the view model type and specifies the UI elements required to refer to an object when displayed on the screen.
View Model
The view model implements the properties and commands that define the functionality of the data displayed on the screen. For I/O operations it uses asynchronous methods and raises events that notify the property changes.
It coordinates the interaction between views and required model classes and sometimes helps modal classes directly bind to the view. ViewModel provides necessary data conversion to make data from the model, easily usable by the view for display.
It implements the INotifyCollectionChanged for ObservableCollection<T> and INotifyPropertyChanged interface. The view model raises a PropertyChanged event when there is a property change, thus helping with two-way data binding.
Model
Model is a self-contained class that includes all the members, properties, validation, and business logic of the properties stored as persistent data in the memory.
We use model classes along with services and repositories that encapsulate data access and caching.
Connecting ViewModels to Views
The data-binding helps with the interaction between the view model and views. The construction of views and view models involves two approaches such as view first composition and view model first composition. Both approaches mainly aim to associate the view with the view model that includes binding context.
View first composition approach includes views that connect to the view models. It helps to create loosely coupled, unit-testable apps and helps ease understanding of the structure. This approach aligns with Microsofts Maui’s navigation system which is responsible for creating pages during navigation.
The view model’s first composition is a complex approach composed of view models with services responsible for locating the view to the view model. It allows the creation of view models from other view models and concentrates on the coding portion besides UI.
Thus the views are completely dependent on the view model for binding the views to the properties in the data source besides referencing view types such as buttons, entries, labels, etc. This makes unit tests possible and reduces software defects.
View Model creation
XAML code to instantiate its respective view model for the view.
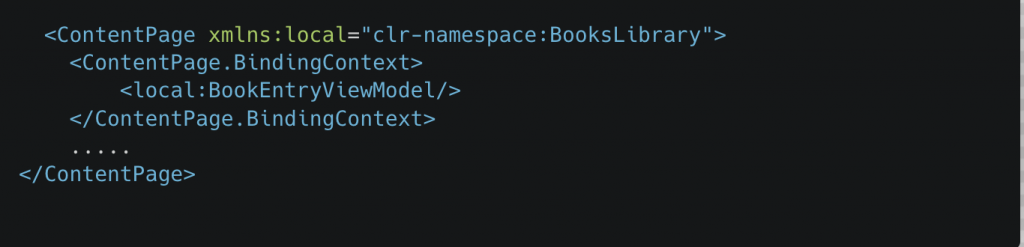
The above way of creation has a disadvantage in that it is suited only for default(parameter-less) constructors, in that case, we can create a view model programmatically.
C# code to create a respective view model
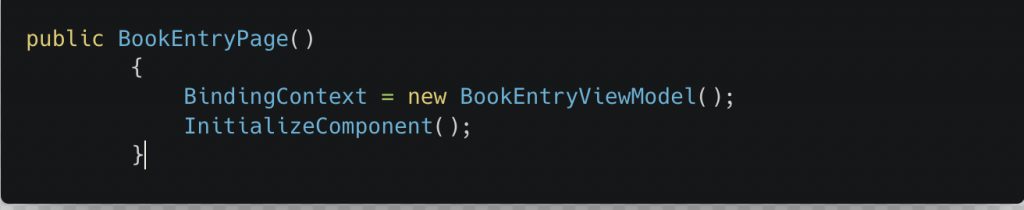
Updating Views
It is necessary to provide change notifications to the controls in the views when the underlying property changes. To notify the change we must implement the INotifyPropertyChanged interface in the view model and model classes.
Following are the important requirements where we must raise the PropertyChanged event.
- If a value of a property is used by other properties in the view model or model.
- At the end of the method which makes the property change.
- Raise the event only after comparing the old and new values are not the same.
- Do not raise an event in the view model constructor since all control initializations will be incomplete.
- Do not raise events in between the loops or during change, raising events after the completion of change is a better practice.
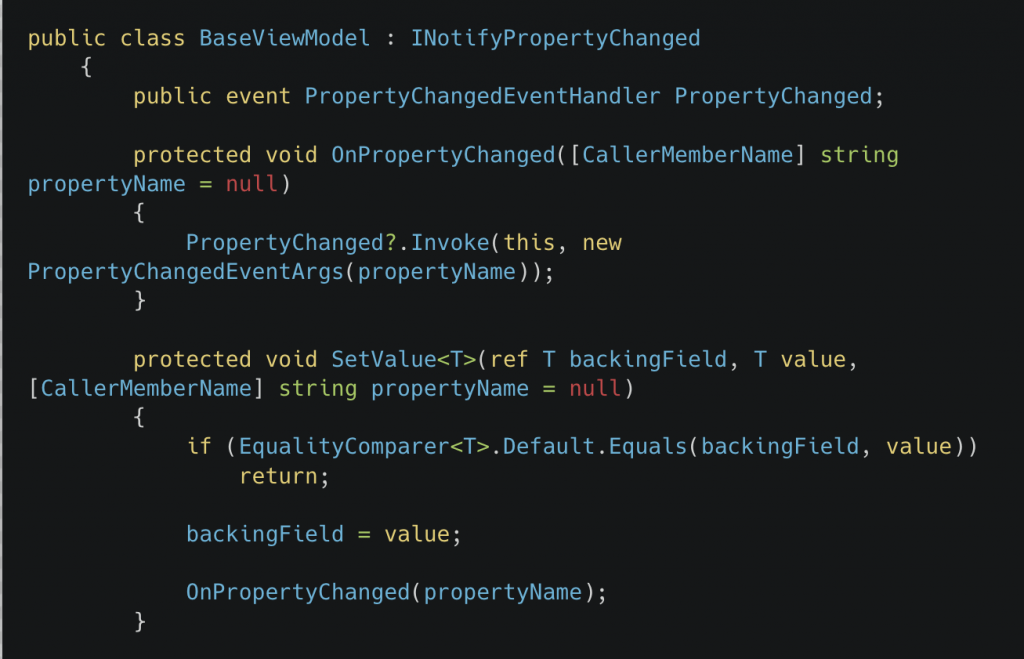
To provide change notifications, a class must implement the INotifyPropertyChanged interface. This interface provides the OnPropertyChanged method which invokes the property change notification.
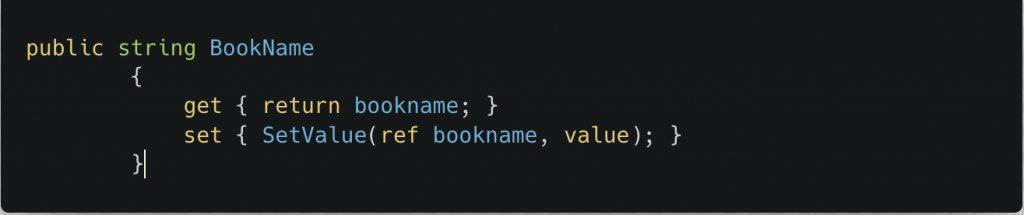
All the classes that need to raise the property changed event can derive the class that implements the INotifyPropertyChanged interface and use the OnPropertyChanged method.
UI interaction using commands
Usually, in the code behind we implement event handlers in response to actions such as a button click which makes tight coupling between design and logic. The main aim of MVVM is to decouple both design and logic so we implement action in ViewModel instead of code-behind.
The command property helps to bind the action with control in the UI which makes decoupling of code and design possible. This makes the view model portable to new platforms.
Implementing commands
The view model which implements the ICommand interface exposes the public properties for binding to the view. One of the commonly used controls such as buttons provides a command property that can be bound to the command object.
C# code
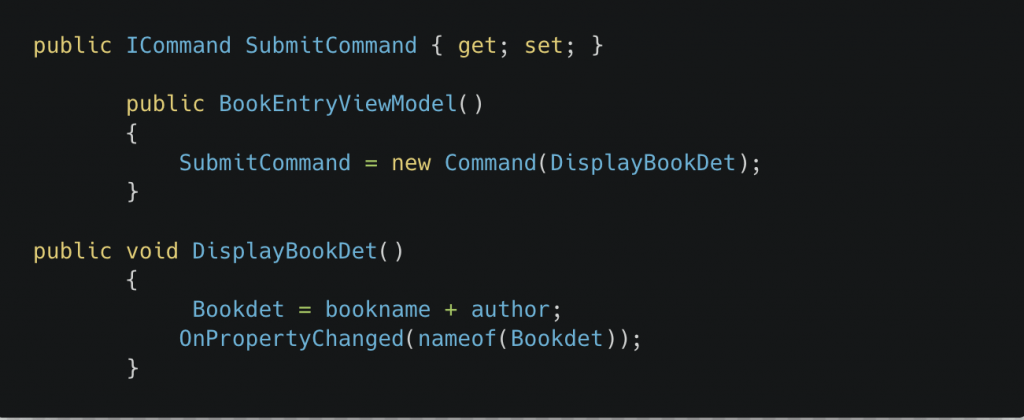
The command with async and await keywords are used to invoke the asynchronous methods which indicate the necessity to await the task completion.
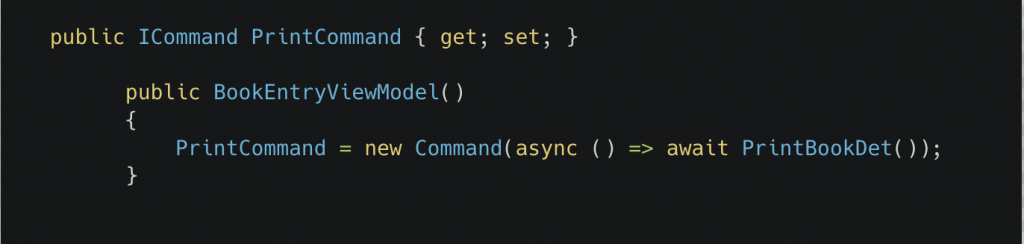
We can pass parameters to the methods of the ICommand interface by mentioning the type to the class which implements the interface.
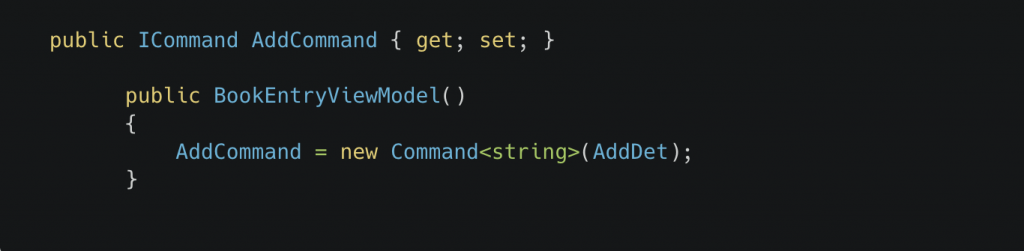
XAML code
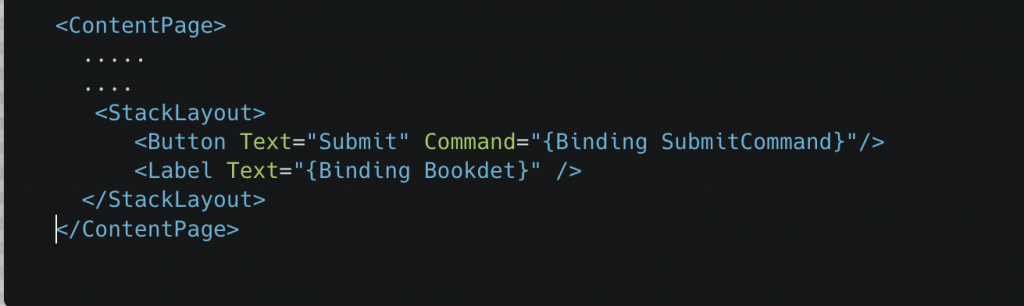
Here we bound a command object such as SubmitCommand to the Command property of the button control. When the user interacts with the control it automatically triggers the target command. It forwards the call to the respective method(DisplayBookDet) mentioned in the view model constructor.