Structure Definition:
The Structure in C is defined as a collection of variables of dissimilar data types, thus
a single structure can contain integer, character, floating-point types,
and pointers as well as arrays.
Each structure element is referred to as a member.
Declaration of Structure in C:
Structure
struct struct-name
{
member1;
member2;
.....
membern;
}structure-variables;
The variables can also be declared separately as
struct struct-name
{
member 1;
member 2;
........
member m;
}
void main()
{
.....
struct struct-name variables;
....
}
Example 1:
struct bike
//bike is the struct-name
{
char name[10],colour[15]; //name ,colour, price and modelno are members
double price;
int modelno;
}c1,c2; // c1 and c2 are structure variables
To access the members of the structure we must use the dot operator.
structure-variable.member-name=value;
for example c1.price=50,000
Anonymous Declaration of Structure in C:
struct
{
float x,y;
}complex;
The above example does not include a structure name, it has the variables x
and y of float type and a structure variable complex. The anonymous
structures are mainly used when there is no need for a tag or structure name
such as in the case of a nested structure.
Example 2:
struct sample
{
int val;
struct
{
int x,y;
}
}var1;
The members of the inner structure do not have a structure name and variable
but it can be accessed using the outer structure variable var1.
Example 3:
struct s
{
int a;
struct
{
float x,y;
}
char arr[10];
}*p;
void main()
{
.....
*p-->x=20;
*p-->y=10;
....
}
*p–> is the reference to the member inside the structure s.
Storage of structure members in memory:
In addition to the knowledge of structure declaration, it is essential to
learn about the storage and alignment of the structure members in the
memory.
To improve performance, avoid memory wastage, and access the memory faster, it
is necessary to understand the alignment of data elements.
The data must be stored at the memory address which is a multiple of the size
with the largest size member first followed by the second-largest.
Let us consider an example
struct Sample
{
char a;
int b;
char c;
}s;
Once the object or variable of a structure is created, a continuous block of
memory is allocated for the structure members. Here we have a char variable
that takes 1byte, integer variable b which takes 4bytes, and
another char that needs another 1byte, so the size of the structure
must be 6bytes(1+4+1) but actually, it will allocate 12byte.
To understand why it allocates 12bytes we must learn about the structure
padding.
Structure Padding
To reduce the number of cycles needed for the processor to read the data,
empty bytes are included in between which is referred to as the structure
padding. The data that can be accessed by the processor at a time is given by
CPU cycle or word size will differ for 32-bit and 64-bit processors.
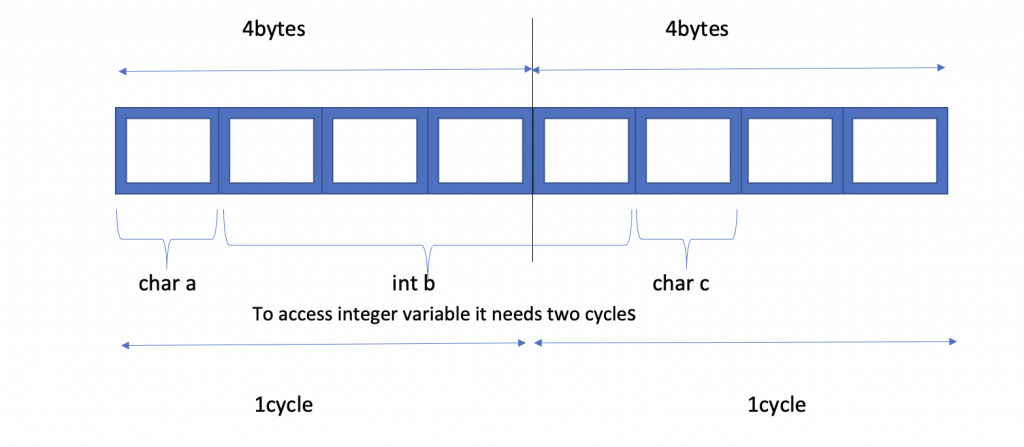
The 32bit processor can access
only 4bytes at a time so word size is 4bytes and
The 64-bit processor can access
only 8bytes at a time and its word size are 8bytes.
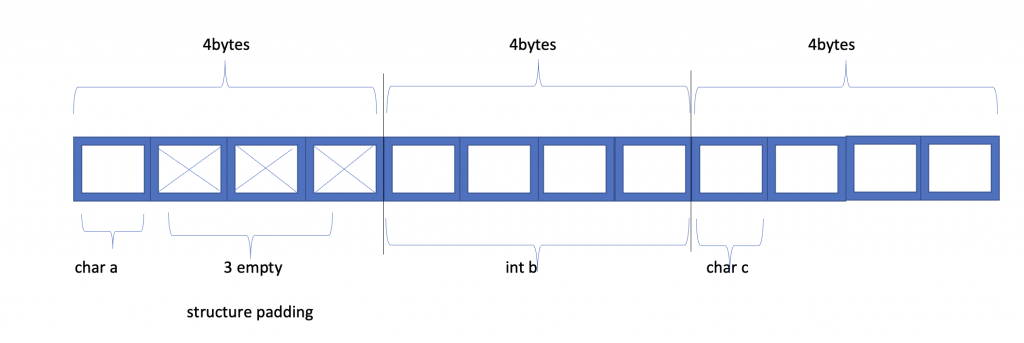
To avoid memory wastage and reduce cycles needed we can declare structure
members in the increasing order of size or we can use structure packing.
This can be done by including a directive
#pragma pack(1) below header files.
After using this structure packing when we check the memory size of the above
sample structure, we will get 6bytes.
Example 4:
Explanation:
In the above example, the structure course has various parameters of
different data types such as int, char, and double, the values for the
character array is initialized using a predefined string
function.
Then each member of the structure such as cname, lang, duration, and fees
are accessed using the structure variable followed by the dot
operator.
Passing structure to a function:
In functions, the values can be passed from the main function to other
functions similarly, the structure variable can be passed to the function.
Example 5:
Explanation:
In this example, we have a structure course that includes variables of
character arrays and the double type and a function GetFeesDet() that
takes the structure variable as an argument.
In the main function, we get input from the user such as cname and
language and the structure variable are passed as an argument. The members
of the structure such as cname and lang are accessed using a structure
variables and compared using string predefined functions.
If it matches with any case then respective fees are assigned else print
“select any of the above-mentioned languages” and course name, language, and
fees.
Bit Fields:
Normally according to the type specified, bytes allocated for the variables
vary, for example, if a variable is declared of type unsigned int, then it
allocates 4bytes of memory.
In some cases, the variables are just used to set status such as True or
False represented by 0, or 1 which just needs 1 bit, in such
cases assigning 4bytes is a waste of memory.
To handle such situations bit fields can be used, which help to define the
width of a variable that represents the number of bytes required.
Syntax:
struct
{
data-type var-name :width
}
Data-type can be an integer, signed integer, or unsigned integer
var-name is the name of the structure member.
width represents the number of bits needed
Example 6:
Explanation:
The width assigned for val is 3bits so when the val is assigned with 5, its
binary value is 101 which can be stored in 3 bits but for 8–>1000, the
first 3bits include 000 which is 0, and for 9–>1001 in which 001 gives
the value 1.
typedef:
It is an alias name for types mainly user-defined data types such as
structure, union, and enum to improve the readability of the code.
For use with predefined types, the typedef can be written as
typedef unsigned int TYPE;
TYPE x,y;
The TYPE is given in uppercase to mention to the user that it is an
abbreviation for typedef unsigned int, it can also be given in
lowercase.
typedef with user-defined data types such as structures
typedef struct calculation
{
int val1,val2;
float result;
}math;
math c1;
c1.val1=10;
c1.val2=20;
The usage of function pointers can be simplified using typedef.
Example 7:
Explanation:
In this example, we have two functions print and printeven each accepts two
arguments
of int type and return type of void, the function pointer for this
function can be simplified by typedef void (*printval)(int *, int).
Since we have given an alias name as printval, instead of assigning like
void (*printval)(int *, int)=print we can assign the function like printval
p=print so we can call the function like p(arr,5) and the respective
function will be executed.
Differences between typedef and #define
An almost similar topic which is always discussed along with typedef is
#define.
typedef:
- It is used to give an alias name for only user-defined or predefined
types. - It is interpreted by the compiler.
- Similar to a statement this must be ended with a semicolon.
#define:
- It is used to give symbolic names for values for eg #define max 100,
#define status True - It is processed by the preprocessor
- It must begin with a # symbol.
Pointer To Structure:
The pointer to structures is mainly used for creating complex data
structures like linked lists, trees, and graphs. The pointer holds the
address of the entire structure and the arrow operator is used to access members.
Syntax:
struct struct-name *ptr;
Example 8:
Explanation:
This program includes a structure named person and we create a variable p1
for that structure and get input from the user such as name, age, and
gender. The address of the structure variable is passed to the displaydet
function.
The value passed inside a function is assigned to pointer variable *p2 and
values are displayed by accessing members using the -> operator.
Array Of Structure in C:
To store and handle the collection of data of different data types, we use
an array of structures such as student details of a college, or school,
employee details of an organization and details of books in a library are
some common examples.
To declare an array of structures, first declare a structure and then we must
declare an array for the structure.
Example 9:
Explanation:
For the structure book, we declare an array det[2] and assign values for
each member of the structure such as name, pages, and price for book1 and
book2.
Then using a for loop all values of book1 and book2 are displayed.
Nested Structure in C:
A structure inside another structure is known as a nested structure,
including multiple members inside a structure will be difficult to
understand so all common features can be grouped under another inner
structure.
Syntax:
struct s1
{
member 1;
member 2;
.......
struct s2
{
member 1;
member 2;
:
:
member n;
}var;
}outervar;
Example 10:
Explanation:
In the nested structure example, we have an outer structure
‘Personaldet‘ which includes a structure ‘Socialaccdet’ in
addition to other members such as name, pin, and location.
The inner structure has members such as platformname and posts and an
object ‘s’ while the outer structure has another object
‘p1’ to access members of the entire structure.
As usual, the outer structure members are accessed using p1 as
p1.name, p1.pin, p1.location, and the inner structure members are
accessed using p1 and s as p1.s.platformname, p1.s.id, and
p1.s.posts, etc.
Union in C
Union in C is defined as a collection of different datatypes in the same memory location. It differs from the structure mainly with the allocation of memory.
Some important differences between structure and union
Structure
- The memory allocated is equal to the total size of the structure members.
- Individual members can be accessed at the same time.
- struct keyword is used.
Union
The memory allocated is equal to the size of the member with large memory size.
Only one member can be accessed at a time since it shares the memory.
union keyword is used.
Defining a union and accessing the members are all same as the structure, the only difference, the union keyword is used.
Defining Union in C:
union union-name
{
member1;
member2;
.....
membern;
}union-variables;
Explanation:
The member of the union with maximum byte size is the char array name[10], but we get 12bytes because of the concept structure padding. If you change that character size to name[8], you will get memory allocated as 8bytes.
Leave your comments about the article and if you have suggestions on future topics, please feel free to mention them.